|
How do you stay on top of your game or get a foothold in WordPress plugin development in 2023? Good news: you don’t need a bunch of flashy tricks up your sleeve to stand out. The best news: we’ve done the hard yards for you and curated a list of essential coding skills, practices, and capabilities to help you succeed in the WordPress ecosystem as a plugin developer.
But what exactly are these coding skills, and where do they stand in 2023? To get the lowdown, I spoke to Freemius founder and CEO Vova Feldman and VP of Engineering Swashata Gosh, who shared 13 must-have coding skills for modern plugin developers.
Starting withâŚ
PHP: The Grand Daddy of WordPress Coding Skills
Out of all the coding skills in WP plugin development, Vova and Swas insist that knowing PHP inside-out remains the alpha and omega.
PHP is the back-end coding language WordPress and its plugins are built on. Iâm reluctant to label it as the most important WP coding skill, but expecting to get into plugin development sans PHP expertise is like signing up for the Tour de France if you can’t ride a bike.
Take note of which version(s) of PHP your WordPress version supports. This helps you gauge if itâs compatible with modern WP products and whether it meets the latest PHP requirements. Currently, WordPress recommends using at least PHP version 7.4. PHP 8 was recently released with the promise of drastically increasing the performance of WordPress websites and web servers.
That said, many themes and plugins are not compatible with PHP 8 (yet). And even though WordPress is backward-compatible, Vova implores that you stay up to date with PHP to take advantage of the new capabilities it offers. âIn time, WordPress will catch up. Since PHP is not fully backward compatible, make sure you donât fall behind because using syntax from older PHP versions may trigger fatal errors on web servers running newer PHP.â
Also, ignoring the PHP/WordPress evolution and neglecting to stay up to speed is detrimental to your coding skills. âThe syntax of PHP is constantly evolving and becoming more strictly typed,â Vova says.
Because itâs a powerful part of WordPress, faulty PHP can easily turn a great product into an unreliable one. Luckily, thereâs an effective way to avoid this potential pothole.
Learn How to Use a PHP Debugger
This is one of the best coding skills to have in your arsenal. Unfortunately â or luckily, depending on where you find yourself â itâs in extremely short supply in our space. Vova expands:
Itâs shocking that a massive ecosystem like WordPress is being built without the use of a back end debugger. Relying on messages being echoed to the screen to understand whatâs going on is highly inefficient.
*cough* Older, somewhat inefficient technology *cough*
The solution? Download (and get to know) Xdebug. Itâs the most common debugger in PHP, and itâll improve your coding skills by allowing you to execute the code step-by-step and inspect the systemâs state in any place, making you 10x more efficient.
Itâs not all smooth sailing, however. Configuring Xdebug with one of PHPâs integrated development environments (or IDEs â more on this later) can be a challenge. Even PhpStorm â which is arguably the best PHP IDE on the market â doesnât offer a preconfigured debugger out of the box.
âYouâll need to do the configuration yourself,â Vova says. âSometimes Xdebug wonât work, and youâll need to play around with it to figure out why. I believe this is the reason many devs donât use it: the transition is perceived as time-consuming. But honestly, they have no idea what theyâre missing out on.â
Learning PHP â and how to use it effectively â is one of the most desirable coding skills in the WP space. But thereâs another WP lingo in town thatâs equally deserving of your attention.
Learn JavaScript Deeply
JavaScript has always been part of WordPressâs DNA, but itâs only come to the fore (pun intended â keep reading 😉) in recent years.
Previously, most of WPâs heavy load was on the back end, which ran on PHP. With the introduction of the Gutenberg visual editor in 2018, much of the business logic in WordPress has moved to the front end. This dynamic shift requires an appropriate application to support it, and Swas champions JavaScript:
Thereâs no better language for this than JavaScript. It can run on servers and clientsâ products, making it truly full-stack.
There are different frameworks built on top to make development more efficient, but JavaScript remains at the core. âItâs the only language developers can code with in the browser, and it needs to be a part of your coding skills repertoire in 2023,â Vova insists.
And speaking of frameworks, donât overlook ReactJS.
ReactJS: The Creme That Crowns JavaScript
ReactJS is an open-source front-end framework written in JavaScript thatâs developed and maintained by Facebook. WordPress has adopted it as a framework because it introduces many modern practices and capabilities that developers can utilize without having to use pure JavaScript.
It handles a lot of magic behind the scenes.
Plugin developers who want to create components that interact with the visual editor â aka Gutenberg â need to know ReactJS. Swas elaborates: âThe library was used to create the new visual editor and â love it or hate it â its future is looking bright. To create powerful blocks, you need inside-out knowledge of ReactJS. Add it to your list of coding skills.â
And on the topic of the visual editorâŚ
Familiarize Yourself With Gutenbergâs APIs
Gutenbergâs APIs allow you to reuse components built with the visual editor and add your own functionalities on top. âTo do this, you need to understand Gutenbergâs functionalities from a coding perspective. This is whatâs meant by knowing the API,â Vova clarifies.
You wonât need to build everything from scratch, which is why this is one of the best time-saving coding skills in WordPress plugin development.
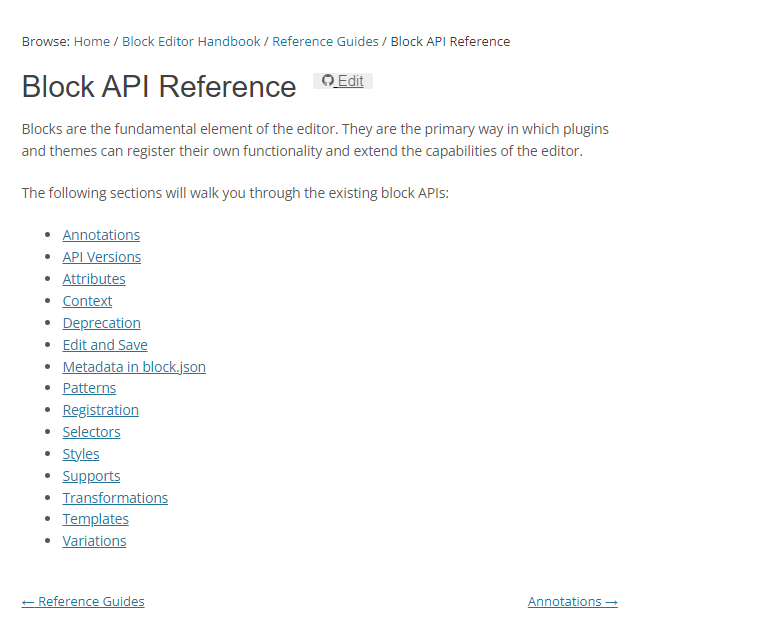
Vova gives an example:
âLetâs say you want to add a block. Thereâs already a built-in function in Gutenberg that allows you to do that, right? You canât change it. But if you want to add a button on top or inside of the block, thereâs something that allows you to do that. The functionality of the button is something youâll have to develop if you want to extend it. But lucky for you, thereâs already an API to communicate this to the editor.â
The visual editor is still a novel addition to our space â itâs nowhere near complete or perfect â but Vova sees great potential:
For now, itâs an unmined area where devs can apply their creativity to extend it, figure out whatâs missing, and innovate from a business perspective.
To echo Swasâs earlier comment: stop worrying and learn to love the block editor instead. Itâs where WordPress is headed, and understanding its APIs is something thatâll bolster your coding skills.
Aside from PHP and JavaScript, there’s a third WP language that’ll help you upgrade your coding skills even further (and it’s probably one you haven’t heard of).
Move On From Vanilla CSS and Onto Sass
WordPress uses CSS (cascading style sheets) to style visuals such as text, images, animations, and transitions. Unfortunately, itâs lacking in two key areas:
- CSS is a rather âdumbâ language. Despite all the advances itâs made, the code itself has not changed much from when it launched.
- CSS is messy. It doesnât have formatting or naming conventions and offers (too) many settings and options, which makes the code akin to the Wild West â almost anyone can do whatever they want with it.
However, CSS ainât leaving WordPress. The workarounds?
- Thereâs a scripting language called Sass, which is essentially a language that is interpreted or compiled into CSS. Vova explains: âIt gives you more capabilities. You can create functions and reusable procedures, and even import files. Thereâs a pre-processor that converts it into traditional CSS. Itâs basically a middleware that allows you to write code thatâs converted into more efficient, smarter, and reusable CSS â like programming rather than styling.â Complementary to Sass, thereâs a library called Compass, which features a large library of pre-built mixins. It offers a simple way to generate image sprites, includes a bunch of typographic functions, helps with cross-browser compatibility, and more.
- Use a convention called BEM (Block Element Modifier). It speeds up the CSS development process by creating a solid structure for the code in the form of CSS structure and naming conventions. Check out this article by CSS-TRICKS to get the lowdown.
Unless your plugin is simple in terms of styling, you need to add Sass and BEM to your collection of coding skills to truly produce something of class (another â very much intended â pun).
A final word on WordPress languages:
Brush Up on the Coding Languages You Already Use
Vova advises:
It depends on your flavor and where you are in your career, but itâs a good idea to take a refresher course roughly every two years or so. New practices and methods will constantly appear as the language evolves. I mentioned that staying on top of PHP is important, but JavaScript and ReactJS are also developing fast â much faster than back end languages.
This is not to say that honing your coding skills requires sacrificing a few months to learn from scratch. But putting a few days aside when it suits your schedule will keep your WP lingo shipshape.
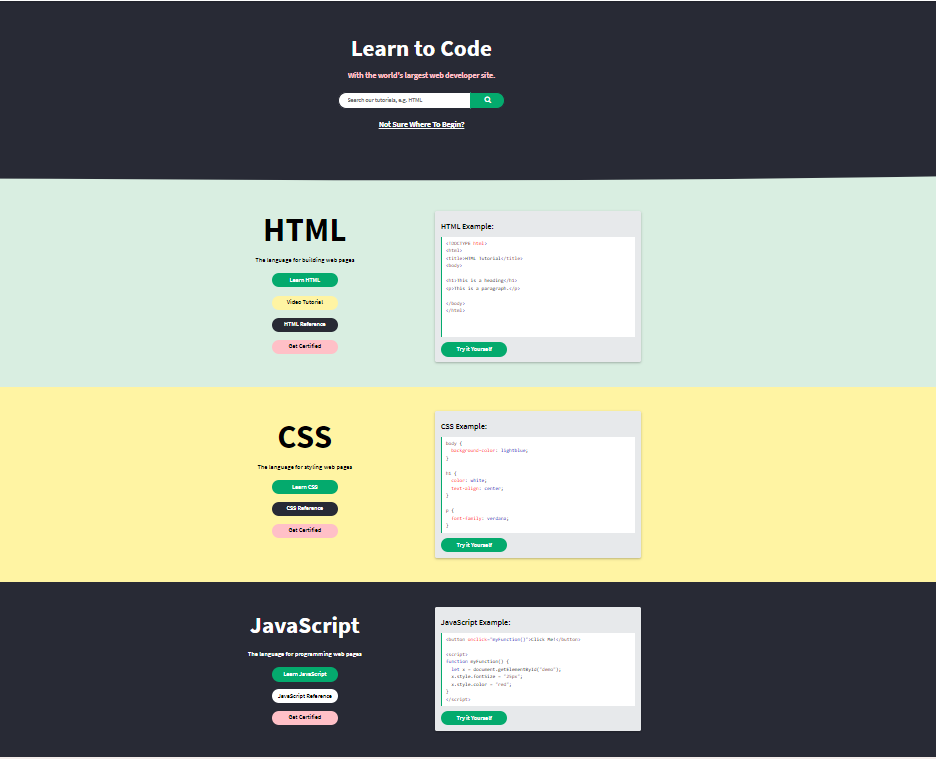
So, what other coding skills are needed to ensure your development engine is well-oiled?
Top Up on Object-Oriented Design (OOD) and Object-Oriented Programming (OOP)
If writing code is like writing a novel, OOD would be the intangible components of the story â exposition, conflict, climax â and OOP would be the physical elements that allow you to follow it: words, sentences, and paragraphs.
Briefly put, OOP is a programming paradigm that uses classes and objects to structure code, while OOD involves (re)applying existing design patterns to construct the final product. Essentially, design patterns are made using OOP. As Swas puts it:
Think of OOP as a toolkit provided by the programming language. The tools, in turn, allow you to implement OOD to solve business problems.
Assuming Iâve cleared up potential confusion 😅 Letâs take a closer look at both of these coding skills.
Object-Oriented Programming
As per TechTarget:
âObject-oriented programming ⌠is a computer programming model that organizes software design around data, or objects, rather than functions and logic. An object can be defined as a data field that has unique attributes and behavior.â
When WordPress was originally developed, it made use of functional coding. Vova elaborates: âThis means that no objects were used; instead, itâs mostly functions-based. While it works okay for the most part, itâs a messy, unorganized, and outdated way to write code.â
In Freemiusâs article â How To Get Hired as a Remote WordPress Developer in 2023 â I note that most WordPress developers are self-taught and many have based their coding skills on the functional code that WordPress is built on. Consequently, their products follow the same structure. This is problematic because it keeps the software in a backward-compatible state while coding languages and practices improve and offer increased functionality.
The WP Core team realized that functional coding is not a practical approach for a large project like WordPress. Following this, WordPress has transitioned significantly to OOP, with PHPâs evolution pushing developers to make the move and up their coding skills by learning it.
âIf youâre not using OOP as a plugin developer in 2023, itâs a problem,â Vova insists.
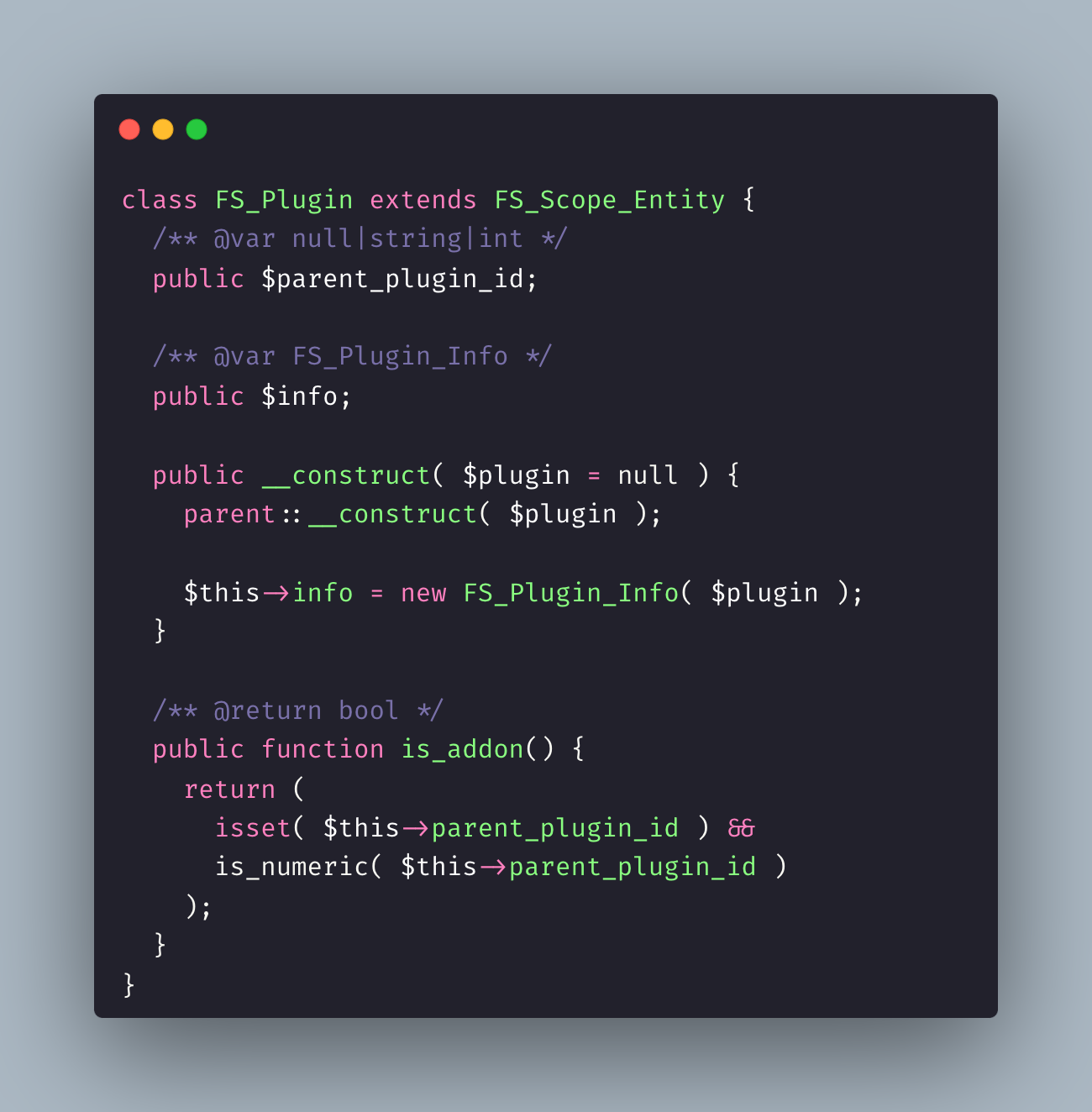
Once youâve got a good grip on OOP, you can use OOD to take your PHP coding skills to new heights.
Object-Oriented Design (OOD)
A definition from Refactoring.Guru:
âDesign patterns are typical solutions to commonly occurring problems in software design. They are like pre-made blueprints that you can customize to solve a recurring design problem in your code.
You canât just find a pattern and copy it into your program, the way you can with off-the-shelf functions or libraries. The pattern is not a specific piece of code, but a general concept for solving a particular problem. You can follow the pattern details and implement a solution that suits the realities of your own program.â
Swas explains:
Letâs say thereâs a security layer in your code. It will comprise many different layers of its own, each with a unique strategy. The layers operate on an algorithm to detect abnormalities. For instance, theyâll pick up on test cards in the checkout and will only allow legitimate ones to pass through.
Suppose a developer were to write all the algorithms and instructions for the security layer in a single function. Think about all the branches and conditions of the logic â it could easily turn into a huge function with 5k to 10k lines of code, having more than three levels of nested if
conditions in many places. Now, consider adding another feature or logic to the function. Not only would it be a mammoth task, but it could very easily break the existing logic, even if done with utmost care.
âYouâd need to solve this big equation by breaking it up into many smaller parts,â Swas says. âPutting them all together gets you to the answer. When we create the equation â or design pattern â weâre establishing a structured way of doing things that we can refer to in future. OOP is the support mechanism that allows us to do this.â
Essentially, OOD helps you structure your pluginâs source code in a scalable way.
You can start adding OOD to your list of coding skills by taking a gander at this comprehensive design pattern mindmap.
Moving along, what other practices should you use to bulletproof your code?
Add Testing
Depending on your objectives, this is initially a catch-22 in terms of coding skills.
A new project is always going to start small. Testing usually gets neglected in the beginning because, at this stage, thereâs not much need for it.
âThere are more testing frameworks available nowadays than a few years ago,â Vova says. âItâll take you a day or two to set one up, which isnât majorly disruptive. However, once you start implementing it, it can easily slow down the project by 10%. And as it grows, this can increase to 20% or even 30%.â
However, weathering this hurdle early on will save you lots of time in the long run. As the project grows, you might want to onboard more people and youâll almost certainly add new features. If you have a framework in place, updates wonât require manual testing.
Swas adds that including both unit and integration testing for your productâs back end and front end is vital. Simply put, unit testing evaluates individual components, while integration testing determines how they work as a whole. Check out this article on CircleCI for a deep dive into the topic.
A few more useful resources:
- This chapter in the WP-CLI Handbook shows you how to run plugin unit and integration tests on various platforms.
- Testing Library and Jest offer effective frameworks for front-end testing.
- Mantle by Alley is a fantastic framework for leveling up your project.
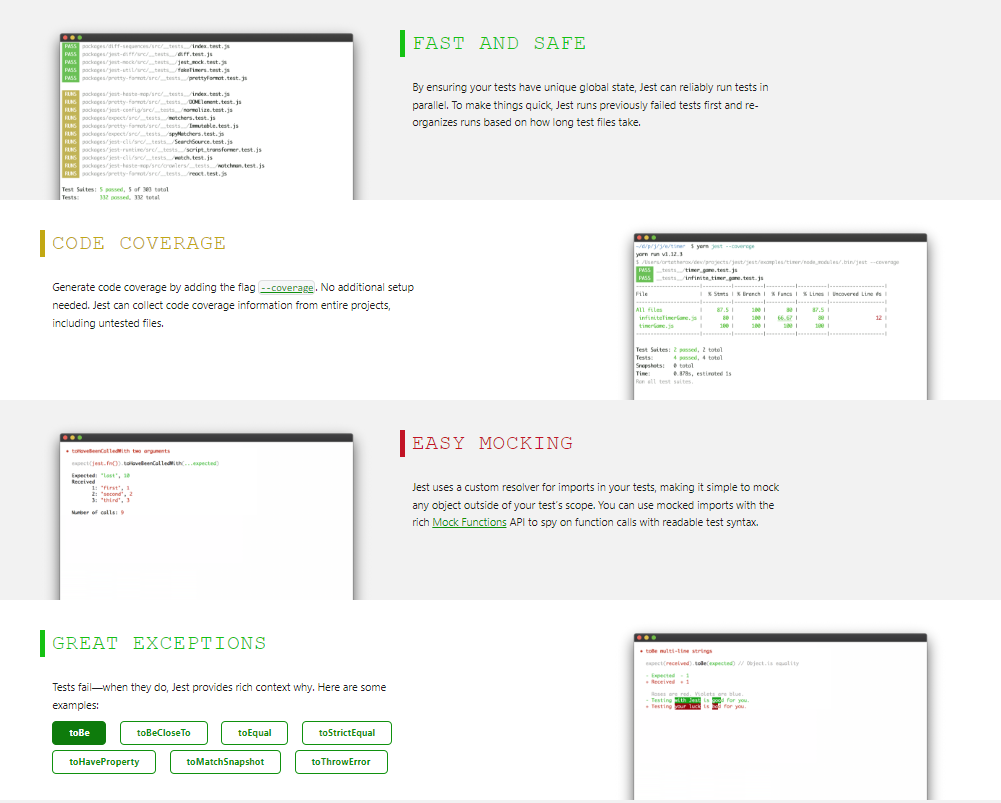
But to begin with, implementing good testing frameworks hinges on being able to write effective code.
Know WordPress Coding Conventions
WordPress coding conventions have to do with naming variables, functions, and classes. Just as there are different conventions for writing different formats â say, an essay versus a diary entry â so too do different software projects and languages have different coding conventions.
âIt helps you navigate through the code and understand whatâs happening,â Vova explains. âSome coding conventions exist because they were implemented by a languageâs creators, but this is usually not the case.â
Different projects will adopt different coding conventions. To illustrate: the coding conventions we use at Freemius are vastly different from WordPressâs, even though both platforms use PHP as the back-end language.
When developing a WordPress product, it makes sense to follow established WordPress coding standards (as documented here). By doing this, youâre making your code âfamiliarâ to other devs who know and follow the same conventions.
It also makes matters easier for a team with multiple developers. Opinions are usually divided on subjects like:
- Whether using camelCase makes more sense than using snake_case.
- If tabs or spaces should be used for indentation.
Following and enforcing pre-determined coding conventions cancels these debates and helps teams focus on more important things like solving business problems.
However, coding conventions arenât only limited to standards. Vova explains:
Developers often say that coding is an art because there are usually 20 different ways of doing one thing, and some methods will be more effective than others. This is where coding conventions come into play. Theyâre useful because they guide you along the projectâs conventional path when you tackle a problem.
This extends to establishing principles for writing software. For example, you might implement a convention in your team dictating that âmagicalâ or âunknownâ numbers may not be used.
Now, letâs say youâre reviewing a line of code that reads as follows:
for ($i = 0; $i < 100; $i++) { $this->indexPostForSearch($i); }
Inevitably, youâd question what the number 100 means, and why it was used.
However, if it was written as followsâŚ
const MAX_ITERATION_FOR_SEARCH_INDEXING = 100; for ($i = 0; $i < MAX_ITERATION_FOR_SEARCH_INDEXING; $i++) { $this->indexPostForSearch($i); }
⌠youâd be able to identify what 100 denotes. For this reason, you should research clean code principles and include them in your coding conventions.
But writing sound code doesnât stop here. As part of your coding skills, you also need to police the conventions you use.
Add a Code Quality Tool
So far, weâve shown why establishing standards and agreeing on principles can help you write better and more consistent code. But as your code and your team grow, youâll realize why using these rules on the fly is not going to cut it. For instance, you might miss something in your code or need to exercise extra caution when reviewing team membersâ pull requests.
Luckily, some tools can review the syntax to ensure whatever changes you or your team have made are following the projectâs conventions. Vova believes itâs one of the best coding skills for productivity.
It can be a huge time-saver for plugin solopreneurs. Ideally, youâll remain consistent and write in the same style. But this is not always the case since conventions evolve. For example, you may find useful conventions in other projects and decide to incorporate them in yours.
But projects with more than one dev can also benefit from code quality tools. âTheyâre useful for reviews,â Vova explains. âIf two people from different backgrounds are working on the same project, theyâll be used to different conventions. A code quality tool robustly verifies that code changes are following the projectâs conventions and can even provide improvement tips. Adding this to your coding skills saves time that wouldâve been allocated to manual code reviews.â
Another reason code quality tools are beneficial for plugin development is to ensure your product follows WordPress standards. All the config files are already out there. Therefore, itâs useful to put a quality assurance tool in place to make sure your code is consistently following conventions.
Apart from formatting standards, code quality tools can also help you detect âsmellsâ in your code. Code that can be written better, is error-prone, or missing some security measures can be detected by these tools. The WordPress Coding Standards tool itself has many linting strategies youâll discover when you start using it.
For PHP and JavaScript, PHP_CodeSniffer and ESLint are the go-to code quality tools used by the broader community.
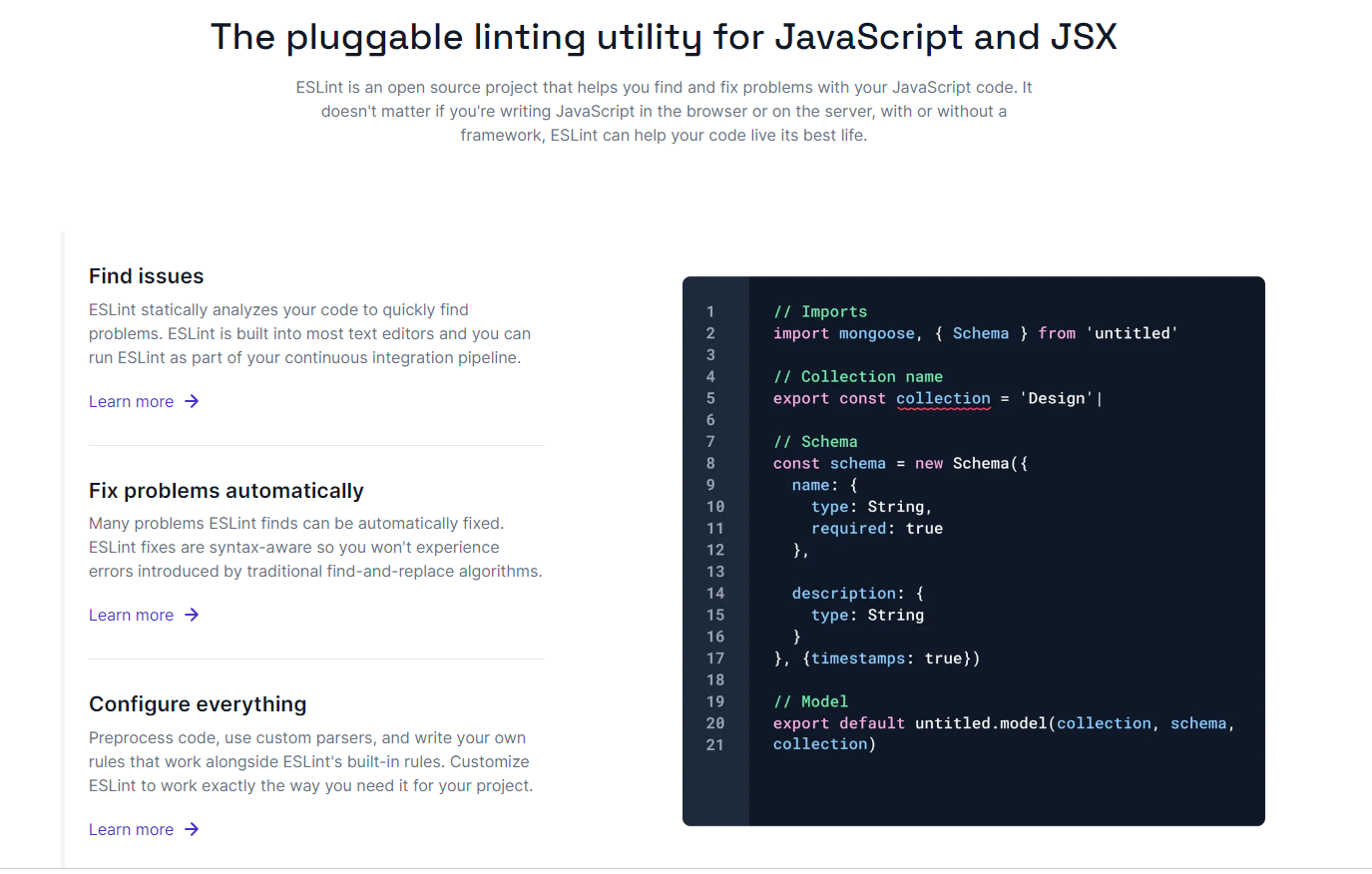
The following packages will help you configure the above tools for WordPress-centric development:
- WordPress Coding Standards (WPCS) for PHP_CodeSniffer
- @wordpress/eslint-plugin for ESLint
Finally, ensure you set up your CI environment to always run tests and check code quality. Research GitHub actions, GitLab pipelines, BitBucket pipelines, and more.
Use a Powerful IDE
Getting to grips with IDEs (integrated development environments) will help enrich your coding skills for many reasons. They can:
- Show you the structure of your code
- Detect and report potential errors, bugs, spelling mistakes, or stylistic issues (including inconsistencies in code conventions)
- Help you execute your code directly from the editor and debug it at the right spot
- Give hints about what the code might do
This article on AWS provides a great definition:
âAn integrated development environment ⌠is a software application that helps programmers develop software code efficiently. It increases developer productivity by combining capabilities such as software editing, building, testing, and packaging in an easy-to-use application. Just as writers use text editors and accountants use spreadsheets, software developers use IDEs to make their job easier.â
âYou can stop the program and inspect the memory,â Swas says. âOn a higher level, developers inspect their codeâs variables and their methods to determine how effective they are.â
He recommends the following IDEs:
- PHPStorm: This IDE offers support for almost all coding languages, including PHP, JavaScript, TypeScript, and CSS.
- Visual Studio Code used in conjunction with the paid Intelephense PHP plugin. âThe JavaScript and TypeScript support is phenomenal,â Swas says.
Use AI
Just like Gutenberg â but at an alarmingly quicker rate â AI has embedded itself in the WP ecosystem. In 2023, you canât afford to leave it out of your collection of coding skills. âIf used correctly, it helps one work more efficiently, allowing you to get the most out of the tools at your disposal,â Vova says. âAs with painting or writing, developers donât actually develop anything at the micro-level. Code is a special combination of functions put together to make something unique. Those functions have been used millions of times before. This is why AI can predict what code you want to write on the micro-level.â
Nevertheless, AI still canât tell what you want to code on a holistic level, which is precisely the reason it isnât a threat to developers (yet 🤐). And, if used correctly, AI can increase your output and help you build even more awesome WordPress products.
Some useful resources:
- This article by ZDNet gives a great breakdown of using ChatGPT to code.
- GitHub Copilot is an excellent AI tool that can be used in your IDE in real-time.
- Amazon CodeWhisperer is also an in-IDE AI tool.
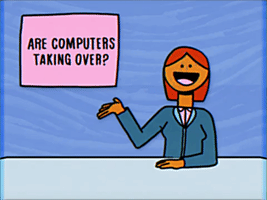
So, between languages, tools, and special know-how, are there any other WordPress coding skills you need to master in 2023?
Keep an Open Mind About Coding Skills
The list of coding skills weâve provided is by no means exhaustive or complete â if anything, itâll be in a state of flux from the moment itâs published.
While some fundamentals will always remain important in WordPress â like code testing and using proper conventions â the space is still super dynamic. Six years ago, almost no one could have predicted how much the block editor would alter the platform. Heck â the AI era only really caught steam about six months ago. On our platform alone, we already have 30+ AI-based plugins selling with Freemius. There are 80 plugins on WordPress.org with GPT in their title, so itâs safe to assume there are probably many more out there.
If thereâs one skill in WordPress thatâll always be valuable, itâs to remain agile enough to ride the constant waves of WordPress change. Letâs enjoy the journey and make cool things while we do 😉
*Feeling inspired by this article? Please get in touch with us! The Freemius team is in a dynamic growth stage, and weâre currently recruiting for the following roles:
Join our team and identify new business opportunities, developing strategic partnerships, and managing key accounts.
Build the core experience and UI of Freemiusâ products, services and dashboards for superior user experience.
Manage the license migration and product integration process for plugin and theme businesses who are starting to sell with Freemius.